As some of you may already know, I've been working on OpenIddict 3.0 for a few months now. One of the main goals of this major release is to merge ASOS (a low-level OpenID Connect server middleware for ASP.NET Core) and OpenIddict (a higher-level OIDC server library designed for less advanced users) into a unified code base, that would ideally represent the best of both worlds.
As part of this task, a new feature was added to OpenIddict: the degraded mode (also known as the ASOS-like or bare mode). Put simply, this mode allows using OpenIddict's server without any backing database. Once enabled, all the features that rely on the OpenIddict application, authorization, scope and token managers (contained in the OpenIddict.Core
package) are automatically disabled, which includes things like client_id
/client_secret
or redirect_uri
validation, reference tokens and token revocation support. In other words, this mode allows switching from an "all you can eat" offer to a "pay-to-play" approach.
A thread, posted on one of the aspnet-contrib repositories gave me a perfect opportunity to showcase this particular feature. The question asked by the commenters was simple: how can I use an external authentication provider like Steam (that implements the legacy OpenID 2.0 protocol) with my own API endpoints?
Steam doesn't issue any access token you could directly use with your API endpoints. Actually, access tokens are not even a thing in OpenID 2.0, which is a pure authentication protocol that doesn't offer any authorization capability (unlike OAuth 1.0/2.0 or OpenID Connect).
So, how do we solve this problem? The most common approach typically consists in creating your own authorization server between your frontend application and the remote authentication provider (here, Steam). This way, when the application needs to authenticate a user, the user is redirected to the authorization server, that delegates the actual authentication part to another party. Once authenticated by that party, the user is redirected back to the main authorization server, that issues an access token to the client application.
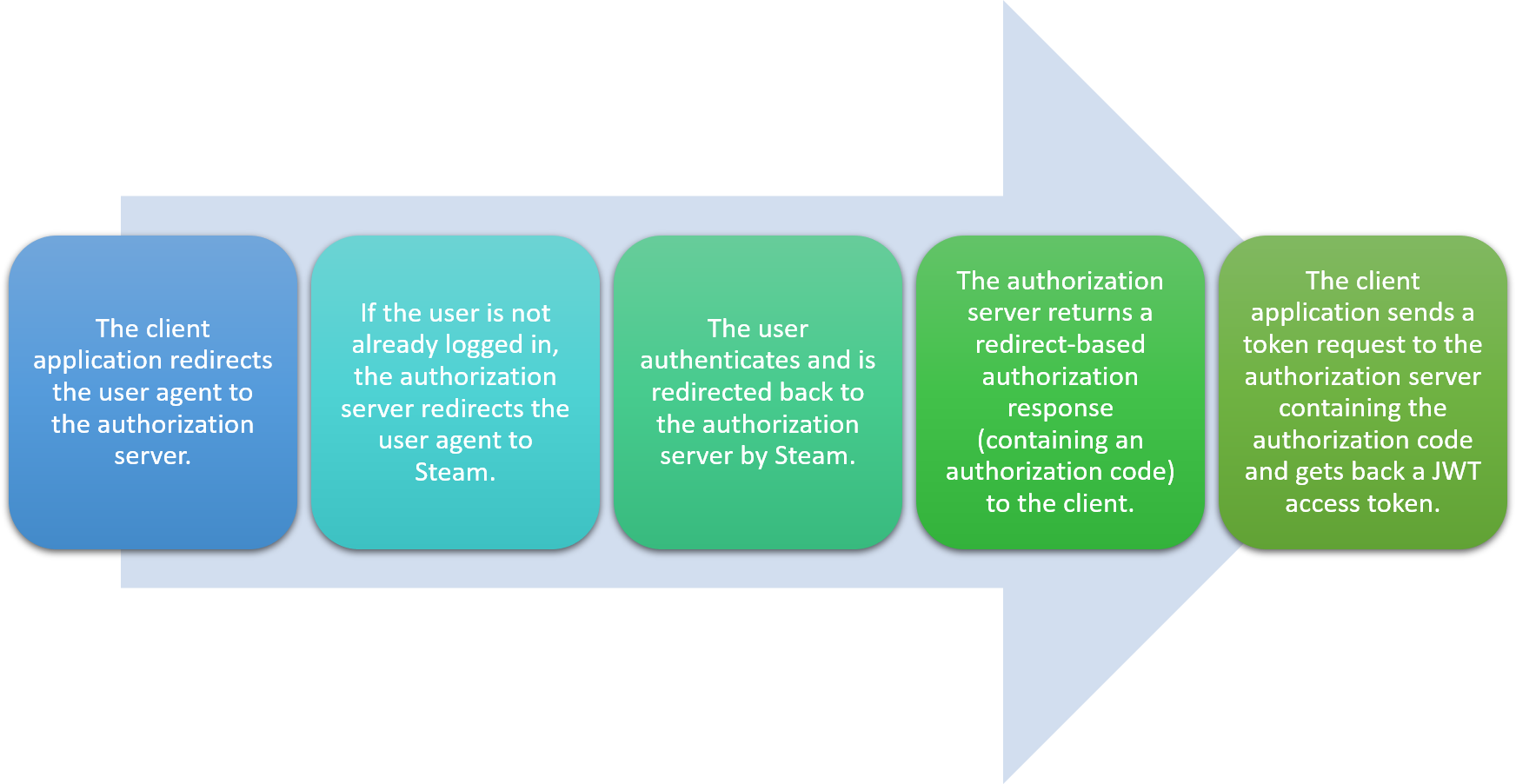
This a super common scenario, that can be implemented using standard protocols like OpenID Connect and well-known implementations like OpenIddict or IdentityServer. However, these options are sometimes considered "overkill" for such simple scenarios. After all, why would you need a fully-fledged OIDC server – with login, registration or consent views – when all you want is to delegate the actual authentication to another server in a totally transparent and almost invisible way?
Rolling your own protocol is tempting... but a very bad idea, as you can't benefit from all the security measures offered by standard flows like OAuth 2.0/OpenID Connect's authorization code flow, whose threat model is clearly identified for many years now. As you may have guessed by now, this is precisely where OpenIddict 3.0's degraded mode can come in handy.